Implementing JWE Authentication in Node.js(Express.js): A Secure Alternative to JWT
Learn how to implement JWE authentication in Express.js and understand why JWE is a secure alternative to JWT for handling sensitive data in web applications.
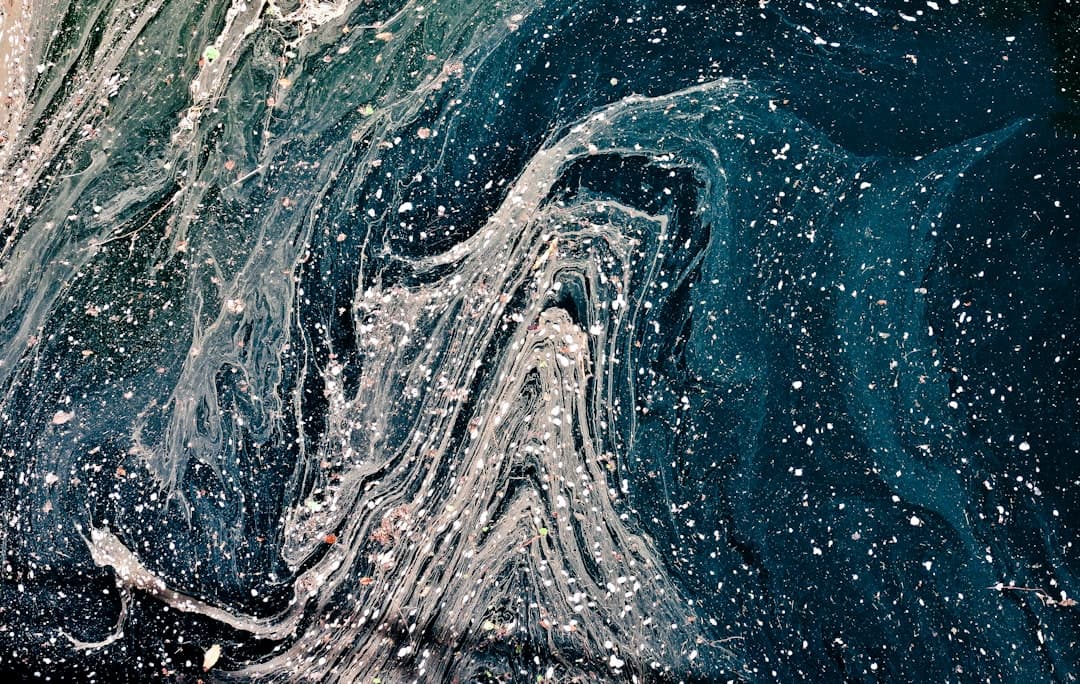
Introduction
Authentication is a critical aspect of web security, and JSON-based token authentication is one of the most widely used methods. While JWT (JSON Web Token) is a common choice, it has limitations in terms of security and data exposure. This is where JWE (JSON Web Encryption) comes in.
In this guide, we will explore how to implement JWE authentication in Express.js and compare it to JWT to show why JWE is a better option for securing sensitive information.
Why JWE is Better Than JWT
What is JWT?
JWT (JSON Web Token) is a compact, URL-safe token format used for transmitting claims between parties. It consists of three parts:
Header: Contains metadata about the token (e.g., algorithm used for signing)
Payload: Contains claims (user data, permissions, etc.)
Signature: Ensures token integrity but does not encrypt data
While JWT is signed to prevent tampering, it is not encrypted, meaning the payload is visible if intercepted.
What is JWE?
JWE (JSON Web Encryption) is an extension of JWT that encrypts the token, preventing unauthorized access to its contents. It consists of five parts:
Header: Specifies encryption algorithms
Encrypted Key: Protects the encryption key
Initialization Vector: Enhances security by introducing randomness
Ciphertext: The encrypted payload
Authentication Tag: Ensures data integrity
Key Differences: JWE vs. JWT
JWT (JSON Web Token) and JWE (JSON Web Encryption) are both used for authentication, but they have key differences in security and data protection. JWT is a compact, signed token that ensures the integrity of the data but does not encrypt it, meaning the payload (which contains user claims) is visible to anyone who intercepts it. This makes JWT suitable for non-sensitive data but risky for applications requiring strict confidentiality. On the other hand, JWE encrypts the entire payload, preventing unauthorized access to its contents. Unlike JWT, which relies solely on a signature to prevent tampering, JWE adds encryption layers, making it more resistant to data exposure, even if intercepted. This enhanced security makes JWE the better choice for handling sensitive information such as personal data, payment details, or confidential user permissions, ensuring compliance with privacy-focused regulations like GDPR and HIPAA.
Installing Required Packages
We need to install the necessary dependencies for implementing JWE in an Express.js application:
npm install express @panva/jose bcryptjs dotenv cors
express: Web framework for Node.js
@panva/jose: Library for JSON Web Encryption (JWE)
bcryptjs: Hashes passwords securely
dotenv: Manages environment variables
cors: Enables cross-origin requests
Implementing JWE Authentication in Express.js
Step 1: User Login & JWE Generation
To authenticate users, we generate a JWE token upon successful login.
const secretKey = createSecretKey(Buffer.from(process.env.JWE_SECRET, "base64"));
const users = [
{ username: "user1", password: "$2a$10$somethingHashed" } // Example user
];
app.post("/login", async (req, res) => {
const { username, password } = req.body;
const user = users.find((u) => u.username === username);
if (!user || !(await bcrypt.compare(password, user.password))) {
return res.status(401).json({ error: "Invalid credentials" });
}
const jwe = await jwtEncrypt({ username }, secretKey, "A256GCM");
res.json({ token: jwe });
});
app.listen(3000, () => console.log("Server running on port 3000"));
Step 2: Middleware for JWE Verification
To secure routes, we create a middleware function to decrypt and verify JWE tokens.
const verifyToken = async (req, res, next) => {
const token = req.headers.authorization?.split(" ")[1];
if (!token) return res.status(403).json({ error: "Access denied" });
try {
const payload = await jwtDecrypt(token, secretKey);
req.user = payload;
next();
} catch (err) {
res.status(401).json({ error: "Invalid token" });
}
};
Step 3: Protecting Routes with JWE
Now, we create a protected route that only authenticated users can access.
app.get("/protected", verifyToken, (req, res) => {
res.json({ message: "This is a protected route", user: req.user });
});
Testing the API
Login to get a JWE token.
Include the token in the Authorization header (
Bearer <token>
) when calling the protected route.If valid, you’ll see the response; otherwise, you’ll get an error.
Best Practices for Secure Authentication
Use strong encryption algorithms (
A256GCM
,RSA-OAEP
)Store secrets in environment variables (
.env
)Implement refresh tokens for better session management
Use role-based access control (RBAC) for different user permissions
Conclusion
JWE provides enhanced security over JWT by encrypting user claims, making it ideal for applications handling sensitive information. While JWT is still useful for public, non-sensitive data, JWE is the better choice when privacy and security are top priorities.
Would you like to learn more about implementing JWE refresh tokens or integrating it with OAuth? Let me know in the comments! 🚀
#JWE encryption
#Web security Express.js
#JSON Web Tokens
#JWT authentication
#Why JWE is better than JWT
#JWE tutorial
#Express.js authentication
#Secure authentication Express.js
#JSON Web Encryption
#JWT authentication Express.js
#JWT authentication Next.js
#nextjs
#nodejs
#expressjs
#JWE vs JWT
#JWE authentication
#Implementing JWE in Express.js