How to implement CUID2 in Next.js 14/15 with Drizzle ORM & Mongoose
Ready to take your Next.js app to the next level? π Learn how to implement CUID2, Drizzle ORM, and Mongoose for a seamless experience in Next.js14/15. Say goodbye to traditional routing and hello to efficiency! #Nextjs #CUID2 #DrizzleORM #Mongoose
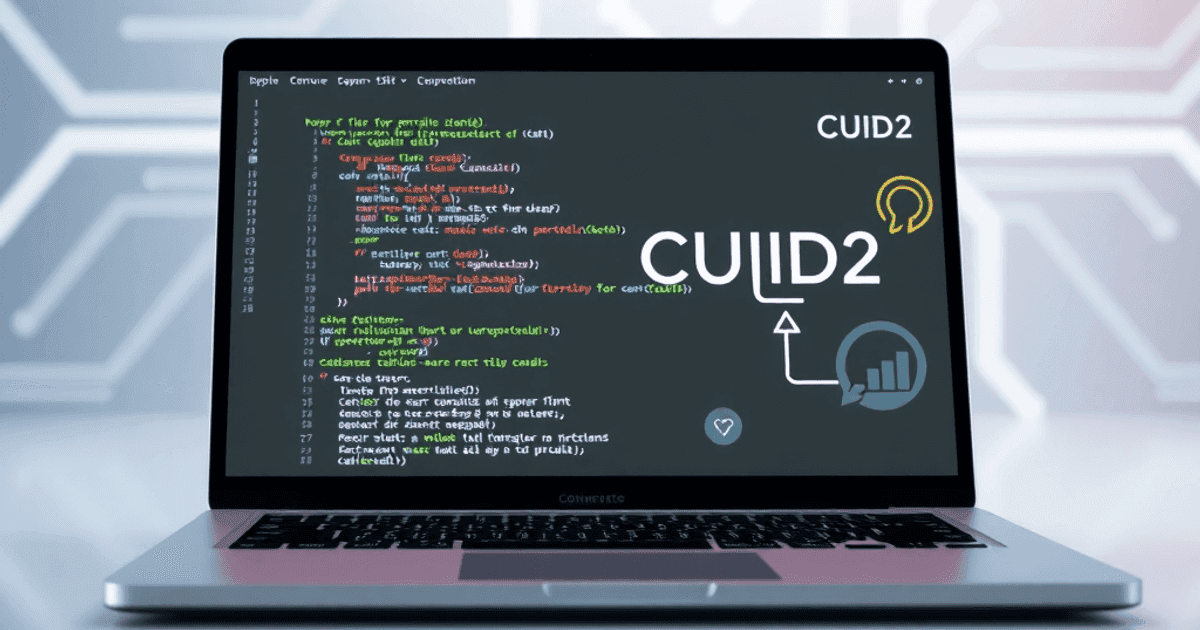
Introduction
In modern web applications, generating unique identifiers is crucial, whether you're working with SQL or NoSQL databases. Traditional methods like auto-incrementing integers and UUIDs have their drawbacksβcollisions, predictability, and inefficiency in distributed systems.
Enter CUID2: a collision-resistant, secure, and human-friendly unique ID generator. In this guide, weβll integrate CUID2 into a Next.js 14/15 project using Drizzle ORM for PostgreSQL and Mongoose for MongoDB.
By the end, youβll be able to generate scalable, secure unique IDs with ease!
Why Choose CUID2 Over UUID?
Before diving into implementation, let's quickly understand why CUID2 is a superior alternative to UUIDs:
β Shorter & More Readable β UUIDs are long and complex, while CUID2 generates compact, human-friendly IDs.
β High Collision Resistance β CUID2 ensures globally unique identifiers, even in distributed environments.
β Crypto-Secure β Uses a cryptographic approach to prevent ID predictability and collisions.
β Efficient for Indexing β UUIDs can cause performance issues in databases due to randomness in indexing. CUID2 minimizes fragmentation and optimizes storage.
Now, letβs implement CUID2 in Next.js 14/15.
Setting Up Your Next.js 14/15 Project
First, install CUID2, Drizzle ORM, and Mongoose:
npm install @paralleldrive/cuid2 drizzle-orm @vercel/postgres mongoose
For database connection, set up PostgreSQL (SQL) and MongoDB (NoSQL) in your project.
Using CUID2 in Next.js API Route (App Router)
Create an API route to generate unique IDs dynamically.
π Create app/api/generate-id/route.js
:
import { NextResponse } from 'next/server';
import { createId } from '@paralleldrive/cuid2';
export function GET() {
return NextResponse.json({ id: createId() });
}
π Test it by visiting /api/generate-id
in your browser. It should return:
{ "id": "clj9t4dmw0001yqz9k6h8w92b" }
Implementing CUID2 in SQL with Drizzle ORM (PostgreSQL)
Drizzle ORM is a lightweight and efficient SQL ORM that pairs well with Next.js App Router.
π Define your schema in db/schema.js
:
import { pgTable, text, varchar } from 'drizzle-orm/pg-core';
import { createId } from '@paralleldrive/cuid2';
export const users = pgTable('users', {
id: text('id').primaryKey().default(() => createId()),
name: varchar('name', { length: 255 }).notNull(),
email: varchar('email', { length: 255 }).unique().notNull()
});
Inserting Data with CUID2
import { db } from '@/db';
import { users } from '@/db/schema';
import { createId } from '@paralleldrive/cuid2';
async function createUser() {
await db.insert(users).values({ id: createId(), name: 'John Doe', email: '[email protected]' });
}
β Now, every new user gets a CUID2-based unique ID!
Implementing CUID2 in NoSQL with Mongoose (MongoDB)
MongoDB, being a document-based database, doesnβt rely on auto-incrementing IDs. Instead, we can use CUID2 for generating unique document identifiers.
π Modify models/User.js
:
import mongoose from 'mongoose';
import { createId } from '@paralleldrive/cuid2';
const UserSchema = new mongoose.Schema({
_id: { type: String, default: createId },
name: String,
email: { type: String, unique: true }
});
export default mongoose.models.User || mongoose.model('User', UserSchema);
Creating a User with CUID2
import dbConnect from '@/lib/dbConnect';
import User from '@/models/User';
async function createUser() {
await dbConnect();
await User.create({ name: 'Jane Doe', email: '[email protected]' });
}
β
Now, every MongoDB document has a secure, collision-resistant CUID2 as _id
.
When to Use CUID2?
π Great for Distributed Systems β No central authority is required to generate unique IDs.
π Ideal for Security-Sensitive Apps β Prevents ID enumeration and brute-force attacks.
π Best for Scalability β Works smoothly across SQL and NoSQL databases.
π Optimized for Performance β Minimizes index fragmentation compared to UUIDs.
Conclusion
CUID2 is a powerful alternative to UUIDs, ensuring secure, human-friendly, and highly scalable unique IDs. With Next.js 14/15 (App Router), it integrates seamlessly into Drizzle ORM for SQL and Mongoose for NoSQL.
By implementing CUID2, your application gains better security, efficiency, and performanceβall while keeping things simple and scalable.
π₯ Ready to future-proof your ID generation? Implement CUID2 today! π
#nextjs
#drizzle
#orm
#cuid2
#nextjs 13
#nextjs 14
#15
#sql
#DrizzleORM
#Mongoose
#vercel
#postgresql
#use cuid in nextjs
#use cuid2 in postgresql
#cuid
#how to use cuid in postgresql