How to Send Emails from a Custom Domain in Node.js (Express.js) with Resend
Learn how to send emails from a custom domain like [email protected] using Express.js and Resend. This step-by-step guide covers setup, API integration, and sending emails efficiently with code examples.
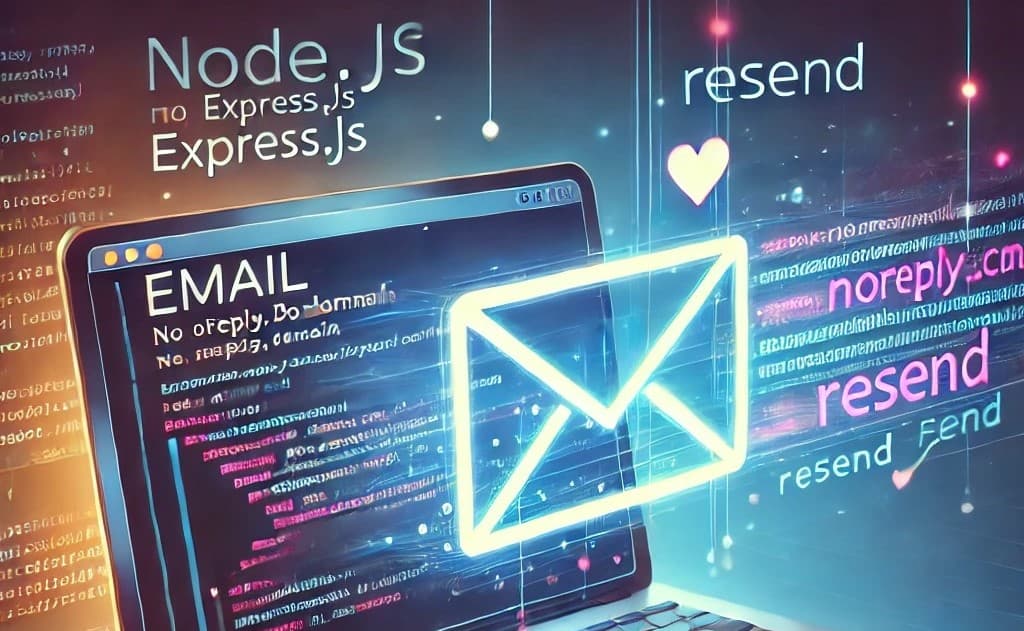
Introduction
Sending emails from a custom domain like [email protected]
is essential for maintaining a professional image and ensuring email deliverability. In this guide, we'll walk through how to send emails in an Express.js project using Resend, a simple and developer-friendly email API.
Prerequisites
Before we start, ensure you have:
A Resend account (sign up here).
A verified domain on Resend.
An Express.js project set up.
Your Resend API key.
Step 1: Install Resend and Express.js
First, install the required dependencies in your Express.js project:
npm install express resend dotenv cors body-parser
Then, create a .env
file to store your API key:
API_RESEND_NOREPLY=your_resend_api_key_here
Step 2: Set Up the Email Sending Function
Create a utility function to send emails using Resend. In utils/email.js
:
import { Resend } from "resend";
import dotenv from "dotenv";
dotenv.config();
const resend = new Resend(process.env.API_RESEND_NOREPLY);
export async function sendMail({ to, subject, html }) {
try {
const response = await resend.emails.send({
from: "[email protected]",
to,
subject,
html,
});
return response;
} catch (error) {
console.error("Email sending error:", error);
throw error;
}
}
Step 3: Create an API Endpoint in Express.js
In server.js
, set up an Express.js server with an endpoint to send emails:
import express from "express";
import cors from "cors";
import bodyParser from "body-parser";
import { sendMail } from "./utils/email.js";
dotenv.config();
const app = express();
const PORT = process.env.PORT || 5000;
app.use(cors());
app.use(bodyParser.json());
app.post("/send-email", async (req, res) => {
const { to, subject, html } = req.body;
if (!to || !subject || !html) {
return res.status(400).json({ error: "Missing required fields" });
}
try {
const emailResponse = await sendMail({ to, subject, html });
return res.status(200).json({ success: true, emailResponse });
} catch (error) {
return res.status(500).json({ error: "Failed to send email" });
}
});
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
Step 4: Test the Email API
You can test your email-sending functionality using Postman or a simple fetch request in a frontend application:
fetch("http://localhost:5000/send-email", {
method: "POST",
headers: { "Content-Type": "application/json" },
body: JSON.stringify({
to: "[email protected]",
subject: "Welcome!",
html: "<p>Hello, welcome to our platform!</p>",
}),
})
.then((res) => res.json())
.then((data) => console.log(data));
Conclusion
Using Resend with Express.js, you can easily send emails from your custom domain in a few simple steps. By following this guide, you ensure better deliverability and a professional email-sending setup. π
If you encounter issues, check Resendβs documentation or verify your domain settings. Happy coding!
FAQs
1. Can I send bulk emails using Resend?
Yes, but it's recommended to follow best practices for email deliverability.
2. How do I track email opens and clicks?
Resend provides analytics to track email performance.
3. Is Resend free to use?
Resend offers a free plan with limited emails per month. Check their pricing for details.
4. How do I avoid spam filters?
Ensure proper SPF, DKIM, and DMARC records are set up in your DNS.
5. Can I use Resend with other frameworks?
Yes! Resend works with Node.js, Express.js, and other backends like Next.js.
#Express.js send email
#Send email with Resend
#resend
#nextjs
#nodejs
#Custom domain email in Express.js
#Resend email API tutorial
#Node.js email sending
#SMTP alternative for Express.js
#How to use Resend in Express
#how to use resend
#Setup email API in Node.js
#Send transactional emails with Resend
#Express.js email integration