How to Modify Cookies from a Next.js 14 Server Component
Learn how to Modify Cookies in a Next.js 14 Server Component easily
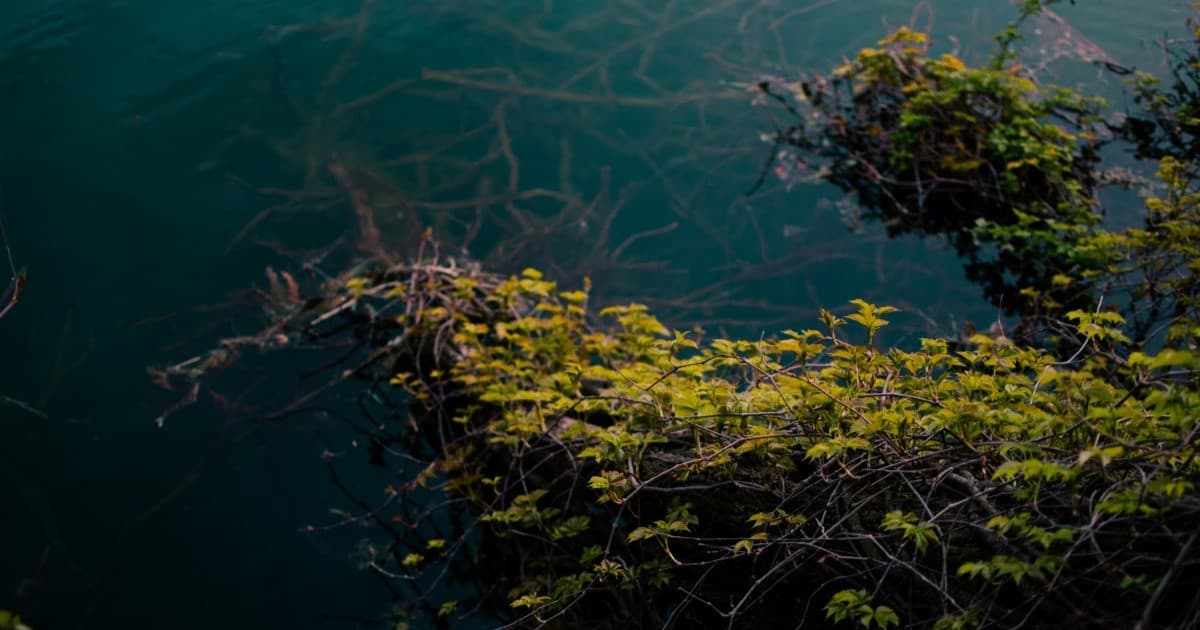
Introduction
In Next.js 14, modifying cookies inside a Server Component is not straightforward. Since Server Components do not have access to the response object, methods like cookies().set()
won’t work as expected.
This guide explains a simple workaround to modify cookies indirectly and provides secure alternatives for handling authentication cookies.
Why Can't You Modify Cookies in a Server Component?
Here’s why modifying cookies directly in a Server Component does not work:
No Access to Response Headers – Server Components don’t interact with HTTP responses, so they cannot modify cookies.
Cookies Are Read-Only in Server Components – You can read cookies using
cookies()
, but you cannot update them.Only the Browser Can Modify Cookies – Since cookies are controlled by the browser, they must be modified via response headers or JavaScript.
To work around this, we need an indirect approach.
Workaround: Modify Cookies Using a Client Component
Since cookies cannot be modified directly in a Server Component, we can:
Read the cookie in a Server Component.
Modify the value on the server.
Pass the modified value to a Client Component.
Update the cookie in the browser using
js-cookie
.
This ensures that the cookie gets updated without breaking Next.js’s architecture.
Step 1: Read and Modify the Cookie in a Server Component
Create a Server Component that reads the cookie and passes the modified value to a Client Component.
import { cookies } from "next/headers";
import ClientComponent from "./ClientComponent";
export default async function Page() {
// Read the cookie on the server
const cookieStore = cookies();
const userToken = cookieStore.get("user-token")?.value || "default-token";
// Modify the cookie value on the server
const updatedToken = `${userToken}-modified`;
return (
<div>
<h1>Server Component</h1>
<p>Original Cookie: {userToken}</p>
<ClientComponent updatedToken={updatedToken} />
</div>
);
}
Step 2: Update the Cookie in a Client Component
Use a Client Component to update the cookie in the browser using js-cookie
.
"use client";
import { useEffect } from "react";
import Cookies from "js-cookie";
export default function ClientComponent({ updatedToken }: { updatedToken: string }) {
useEffect(() => {
// Set the updated cookie in the browser
Cookies.set("user-token", updatedToken, { path: "/" });
}, [updatedToken]);
return (
<div>
<h2>Client Component</h2>
<p>New Token Set in Browser: {updatedToken}</p>
</div>
);
}
Now, when the Client Component mounts, it updates the cookie in the browser.
Downsides of This Approach
While this method works, it has some limitations:
No HTTP-Only Cookies – Cookies set with
js-cookie
can be accessed by JavaScript, making them unsuitable for authentication.Security Risks – Client-side cookies are vulnerable to XSS attacks.
Delayed Cookie Updates – Cookies are only updated after the Client Component mounts.
If security is a concern, consider using a more secure method.
Secure Alternatives for HTTP-Only Cookies
For secure authentication, use API routes or Server Actions instead of js-cookie
.
Option 1: Use an API Route to Set Secure Cookies
This method sets an HTTP-only cookie in the response headers.
import { NextResponse } from "next/server";
export async function GET() {
const response = new NextResponse("Cookie Set", { status: 200 });
response.cookies.set("user-token", "123456", {
httpOnly: true,
secure: true,
path: "/",
});
return response;
}
✅ Secure: The cookie is HTTP-only and cannot be accessed by JavaScript.
❌ Requires an API call to update cookies.
Option 2: Use Server Actions (Experimental)
Next.js Server Actions allow modifying cookies directly on the server.
"use server";
import { cookies } from "next/headers";
import { redirect } from "next/navigation";
export async function setUserCookie() {
cookies().set("user-token", "abcdef", { httpOnly: true, secure: true });
redirect("/dashboard"); // Redirect after setting the cookie
}
✅ Secure: Does not require client-side JavaScript.
❌ Experimental: This feature may change in future Next.js updates.
Conclusion
If you don’t need HTTP-only cookies, the Client Component workaround can help update cookies indirectly. However, for secure authentication, use an API route or Server Actions to set HTTP-only cookies.
Choose the best method based on your security and performance needs.
#nextjs
#server component
#modify cookie